How to get the information about the Laravel batches like its name, progress, status, failed count, current completion count, etc? You can use below methods on batch
//Get Batch Id
$batch->id;
// Get Batch name if given by you
$batch->name;
// Total number of job instance in the batch
$batch->totalJobs;
// Total number of remaining jobs
$batch->pendingJobs;
// Total number of failed jobs
$batch->failedJobs;
// Total number of completed jobs
$batch->processedJobs();
// progress as a pecentage : 0-100%
$batch->progress();
// Return true when batch completed.
$batch->finished();
// Return true if batch execution is cancelled.
$batch->cancelled();
Full Example
First create a sample job class called PrepareMailCampaign using below artisan command.
php artisan make:job PrepareMailCampaign
Open PrepareMailCampaign job class and add Batchable trait.
<?php
namespace App\Jobs;
use Illuminate\Bus\Batchable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Queue\Queueable;
use Illuminate\Support\Facades\Log;
class PrepareMailCampaign implements ShouldQueue
{
use Queueable, Batchable;
/**
* Create a new job instance.
*/
public function __construct()
{
//
}
/**
* Execute the job.
*/
public function handle(): void
{
}
}
Now open web.php file and add below code.
- Root route – create Laravel batch and execute
- Create array of products
- Create empty array to hold array of PrepareMailCampaign job instances.
- Iterate products array and create PrepareMailCampaign job instances array.
- Dispatch the Laravel batch.
- Send link to access batch data.
- Batch id route
- Get batch from the batch id
- show batch data in a table.
use App\Jobs\PrepareMailCampaign;
use Illuminate\Support\Facades\Bus;
use Illuminate\Support\Facades\Log;
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
//Log request
Log::info("Received a get request on root route");
//List of products that require main campaigns.
$products = ['prod_1', 'prod_2', 'prod_3', 'prod_4', 'prod_5'];
//Create empty batch array to hold prepareMainCampaign jobs
$mailCampaignJobBatch = [];
//Create the mainCampaign Batch
foreach($products as $product)
{
array_push($mailCampaignJobBatch, new PrepareMailCampaign($product));
}
//Dispatch the batch
$batch = Bus::batch($mailCampaignJobBatch)->name('Mail Campaign Batch')->dispatch();
echo "<a href='/{$batch->id}'>Get Batch information</a>";
});
Route::get('/{batchId}', function ($batchId) {
$batch = Bus::findBatch($batchId);
echo "<table border='1' style='border-collapse: collapse; width: 450px;'>
<thead>
<tr>
<th>Key</th>
<th>Value</th>
</tr>
</thead>
<tbody>
<tr>
<td>Batch ID</td>
<td>{$batch->id}</td>
</tr>
<tr>
<td>Batch Name</td>
<td>{$batch->name}</td>
</tr>
<tr>
<td>Total Number of Jobs</td>
<td>{$batch->totalJobs}</td>
</tr>
<tr>
<td>Completed Jobs</td>
<td>{$batch->processedJobs()}</td>
</tr>
<tr>
<td>Remaining Jobs</td>
<td>{$batch->pendingJobs}</td>
</tr>
<tr>
<td>Failed Jobs</td>
<td>{$batch->failedJobs}</td>
</tr>
<tr>
<td>Completion Percentage</td>
<td>{$batch->progress()}%</td>
</tr>
<tr>
<td>Is Batch Completed?</td>
<td>{$batch->finished()}</td>
</tr>
<tr>
<td>Is Batch Cancelled?</td>
<td>{$batch->cancelled()}</td>
</tr>
</tbody>
</table>";
});
Run a queue worker using below artisan command
php artisan queue:work
Load root route of this Laravel web application using your web browser. You will “Get Batch infomation” link. Click on it. Refresh it to see current status of the ongoing batch. You will see something like below gif.
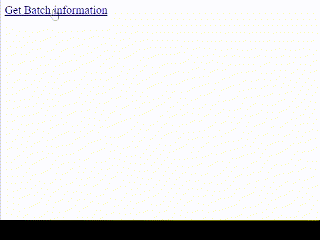